Ultrasonic is a great sensor when it comes to measuring a distance of an object. Ultrasonic sensors are based on measuring the properties of sound waves. It has a transmitter/trigger to generate a sound pulse and a receiver to receive an echo pulse coming from the targeted object. By calculating the traveling time of trigger pulse and echo pulse we can easily calculate the distance between object and sensor. As the speed of the sound is already known to us, we can easily calculate the distance. Arduino Distance Measurement using Ultrasonic Sensor.
In this project, we are using an ultrasonic sensor to measure the distance of an object from the sensor and an LCD display to see the distance.
You will need :
- Arduino
- HC-SR04 Ultrasonic Sensor Module
- 16×2 LCD
- Breadboard
- Jumper wire
- DC power supply or battery
HC-SR04 Ultrasonic Sensor Module :
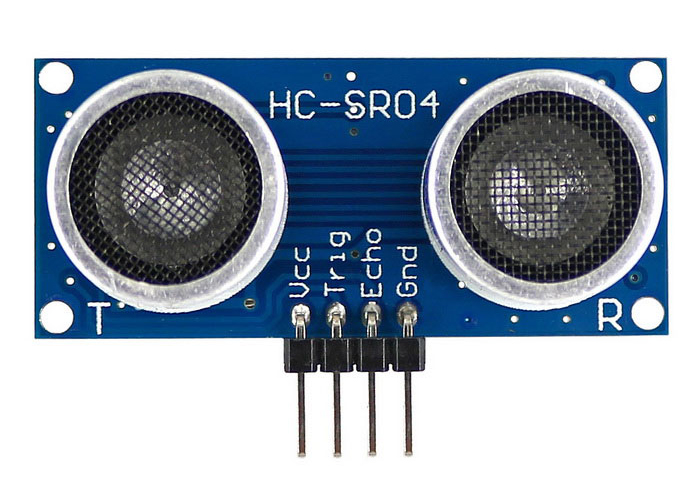
This is an HC-SR04 ultrasonic sensor. This sensor can provide 2cm to 400cm of non-contact measurement functionality with an accuracy of up to 3mm. Each HC-SR04 module includes an ultrasonic transmitter, a receiver, and a control circuit.
Working Principle of Ultrasonic Sensor :
Ultrasonic sensors emit short, high-frequency pulses at regular intervals. These propagate within the air at the velocity of sound. If they strike an object, then they’re reflected back as echo signals to the sensor. It computes the time-span between emitting the signal and receiving the echo itself.
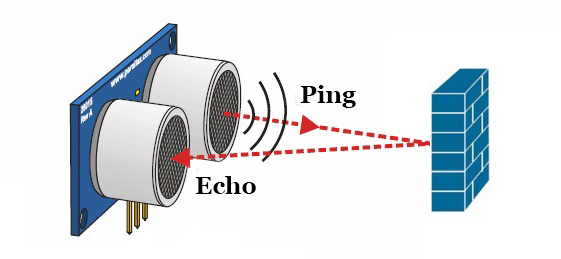
And as we know the speed of the sound wave we can easily calculate the distance between the sensor and object.
As we can see in the picture there are four pins – Ground, VCC, Trigger, and Echo. VCC and ground pins are for the power supply. The trigger pin is for creating trigger pulse and the Echo pin is for receiving echo pulse.
In order to generate the trigger pulse, you need to set the Trigger pin on a High State for 10 µs. An ultrasonic sensor automatically sends 8 cycles of 40 kHz ultrasound signal and checks for its echo. When it receives an echo signal it automatically computes the time-span between emitting the signal and receiving the echo itself.
And as we know the speed of the sound wave we can easily calculate the distance between the sensor and object.
As we can see in the picture there are four pins – Ground, VCC, Trigger, and Echo. VCC and ground pins are for the power supply. The trigger pin is for creating a trigger pulse and the Echo pin is for receiving an echo pulse.
In order to generate the trigger pulse, you need to set the Trigger pin on a High State for 10 µs. An ultrasonic sensor automatically sends 8 cycles of 40 kHz ultrasound signal and checks for its echo. When it receives an echo signal it automatically computes the time-span between emitting the signal and receiving the echo itself.
Circuit Diagram :
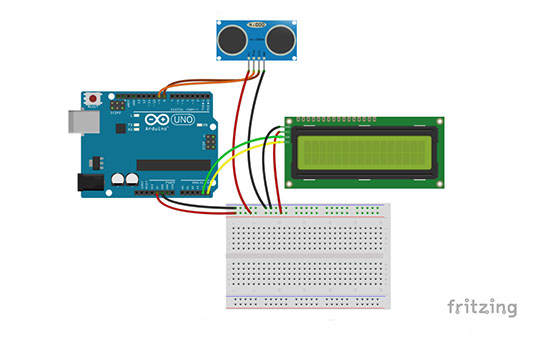
Here we are using an HC-SR04 Ultrasonic Sensor module, an LCD I2C display to Show the distance, and Arduino Uno for controlling the sensor and LCD display.
In the above circuit diagram, you can clearly see that the Ultrasonic echo pin and trigger pin are directly connected to Arduino pin no 8 and 9 respectively. LCD SCL and SDA pins are connected to Arduino SCL and SDA pins respectively. Ultrasonic VCC pin and LCD VCC pin are connected to Arduino 5v pin via a breadboard. Ultrasonic ground pin and LCD ground pin are connected to Arduino ground pin via a breadboard.
Note :- In Arduino Uno SCL pin is A5 and the SDA pin is A4.
Arduino Code
/*
* Arduino Distance Measurement using Ultrasonic Sensor
* Crated by Kishor Mahata
* www.circuitgeeks.com
*
* Pin config
* echo pin >> arduino pin 8
* trigger pin >> arduino pin 9
* ground pin >> arduino ground pin
* vcc pin >> arduino vcc pin
* lcd SCL pin >> arduino SCL pin, in case of arduino uno this is pin A5
* lcd SDA pin >> arduino SDA pin, in case of arduino uno this is pin A4
* lcd ground >> arduino ground
* lcd vcc >> arduino 5v pin
*/
#include <Wire.h>
#include "LiquidCrystal_I2C.h"
#define trig 9 //define trigger pin at arduino pin 9.
#define echo 8 //define echo pin at arduino pin 8.
LiquidCrystal_I2C lcd(0x27, 16, 2); // Set the LCD address to 0x27 for a 16 chars and 2 line display
// defines variables
float duration,dist_inch;
int dist_cm;
void setup() {
Serial.begin(9600); // initialize serial communications at 9600 bps.
lcd.clear();
lcd.backlight(); // Turn on the blacklight and print a message.
lcd.begin(); // initialize the LCD
lcd.setCursor(0, 0); // Set the cursor to column 0,row 0.
lcd.print("dist. cm :"); // Print the massage
lcd.setCursor(0, 1); // Set the cursor to column 0,row 1.
lcd.print("dist. inch:"); // Print the massage
}
void loop() {
// Clears the trigPin
digitalWrite(trig, LOW);
delayMicroseconds(2);
// Sets the trigPin on HIGH state for 10 micro seconds
digitalWrite(trig, HIGH);
delayMicroseconds(10);
digitalWrite(trig, LOW);
// Reads the echoPin
duration = pulseIn(echo, HIGH);
// Calculating the distance
dist_cm = duration/58;
dist_inch = dist_cm/2.54;
// Prints the distance on the LCD Display
lcd.setCursor(11, 0);
lcd.print(dist_cm); // Prints the distance in cm
lcd.setCursor(11, 1);
lcd.print(dist_inch); // Prints the distance in meter
delay(500);
// Clear the LCD
lcd.setCursor(11, 0);
lcd.print(" ");
lcd.setCursor(11, 1);
lcd.print(" ");
delay(10); // wait 10 milliseconds before the next loop
}
Explaining the Code :
In the first portion of the program, we include the necessary header file, define pin and variables, and Set the LCD address to 0x27 for 16 chars and 2 line display. In the setup section, we initialize serial communications at 9600 bps. Then we initialize the LCD and turn on the backlight and print a message which is fixed. In the void loop section, we generate a trigger pulse at the trigger pin and read the echo at the echo pin. Then we calculate the distance in cm and meter and print it on the LCD. We set delay 500 milliseconds so we get updated distance an interval of 1/2 second. In the last portion, we clear the LCD buffer.
I hope you enjoyed this tutorial and learned something new. Feel free to ask any questions in the comment section below.