An OLED display is the best alternative to the character LCD. The OLED display looks very cool because of its thin design and high-contrast screen.
In this Arduino OLED display tutorial, I will show you how to connect an OLED display with an Arduino and draw common shapes on it. How to draw custom graphics, scrolling text on the display, etc.
Table of Contents
- 1 OLED Display
- 2 SSD1306 OLED Driver
- 3 Power Requirement for SSD1306 OLED
- 4 Memory Mapping
- 5 OLED Display Pinout
- 6 Arduino OLED Connection
- 7 Installing Libraries for OLED Display
- 8 Common Functions and Their Parameters
- 9 Testing the OLED
- 10 Printing Text & Numbers
- 11 Draw Different Shapes (Circle, Rectangle, Triangle, etc)
- 12 Invert The Display
- 13 Inverted Text and Numbers
- 14 Using Different Fonts from The Library
- 15 Arduino OLED Scrolling Text
- 16 Draw ASCII Characters
- 17 Display Custom Graphics (Bitmap Image)
- 18 Conclusions
OLED Display
Organic Light-Emitting Diode or OLED Display is made with sheets of organic material that emit light in response to an electric current. This sheet is placed between two electrodes and at least one electrode needs to be transparent. So that we could see the light coming from the display.
OLED display comes in different shapes like 128×64, 128×32, 64×64, etc. In this tutorial, I will use a 128×64 pixel blue OLED display with an Arduino UNO board.
SSD1306 OLED Driver
There are various different display controllers available in the market – SSD1306, SSD1327, SH1106, etc. among them SSD1306 is the most popular. The SSD1306 controller has an internal RAM of 128×64 pixels.
It can communicate using both I2C and SPI protocols. I2C protocol only needs 2 pins to communicate with a microcontroller whereas SPI protocol requires a minimum of 5 to 6 pins. But SPI is faster than I2C. So you have to select between speed and pins according to your project’s needs.
Power Requirement for SSD1306 OLED
The SSD1306 OLED driver requires 1.65v to 3.3v whereas the display needs 7v to 15v. To meet these two different power requirements there is an onboard voltage regulator which takes an input voltage from 1.8v and 6v and provides a stable 3.3v output voltage. And a charge pump circuit to obtain a higher voltage for the display. So you don’t need any extra power circuitry.
A regular 0.96-inch OLED display requires a 3.3v to 5v power supply and uses about 20mA current.
Memory Mapping
The SSD1306 controller has a bit-mapped static RAM called GDDRAM ( Graphic Display Data RAM ). The size of the RAM is 128 x 64 bits (8,192 bits or 1KB). It holds the bit pattern to be displayed on the screen. This 1KB memory is divided into eight pages, from PAGE0 to PAGE7, which are used for monochrome 128×64 dot matrix displays.
Each page contains 128 columns/segments (blocks 0 to 127). And each column can store 8 bits of data (from 0 to 7).
OLED Display Pinout
Here you can see the picture of an I2C OLED display. It has only four pins. From the picture, you can see that the left two pins are ground and VCC and the other two pins are SCL or SCK, and SDA.
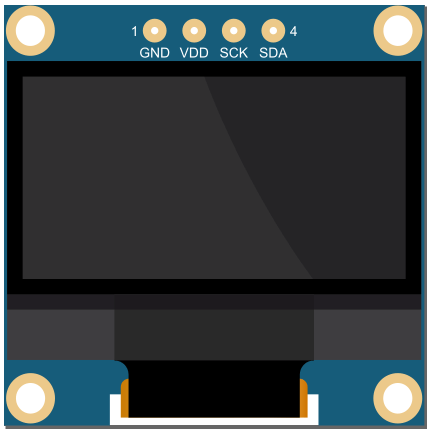
Arduino OLED Connection
An I2C OLED display has 4 pins VSS, Ground, SCL, and SDA. The display needs 3.3v to 5v to operate. So connect the VSS pin to the Arduino 5v pin and ground to the Arduino ground.
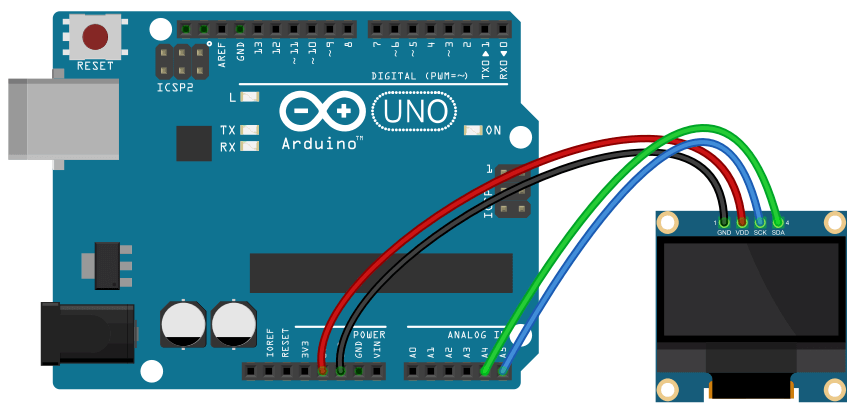
If you are using a different Arduino board, check the correct SCL and SDA pin for that board.
- Arduino UNO : SCL > A5, SDA > A4
- Arduino Nano : SCL > A5, SDA > A4
- Arduino Pro Mini : SCL > A5, SDA > A4
- Arduino Mega : SCL > 21, SDA > 20
- Arduino Leonardo : SCL > 21, SDA > 20
Installing Libraries for OLED Display
To control an OLED with an SSD1306 driver we need two libraries. Adafruit SSD1306 and the adafruit GFX.
To install these libraries navigate to Tools > Manage Libraries
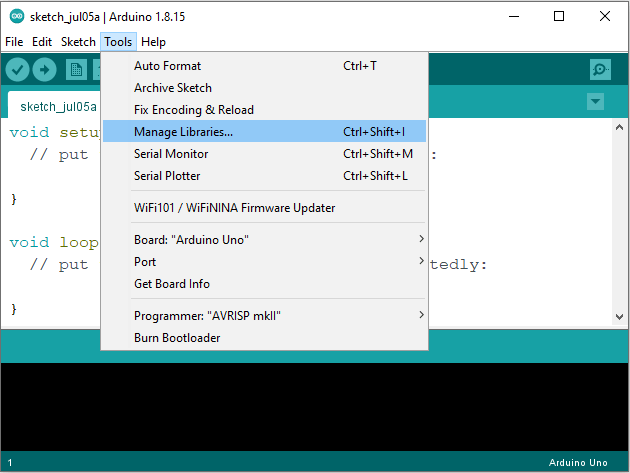
Wait for the screen to load properly. Then type “Adafruit SSD1306” in the search box. You will find a couple of entries there. Look for Adafruit SSD1306 and select install.
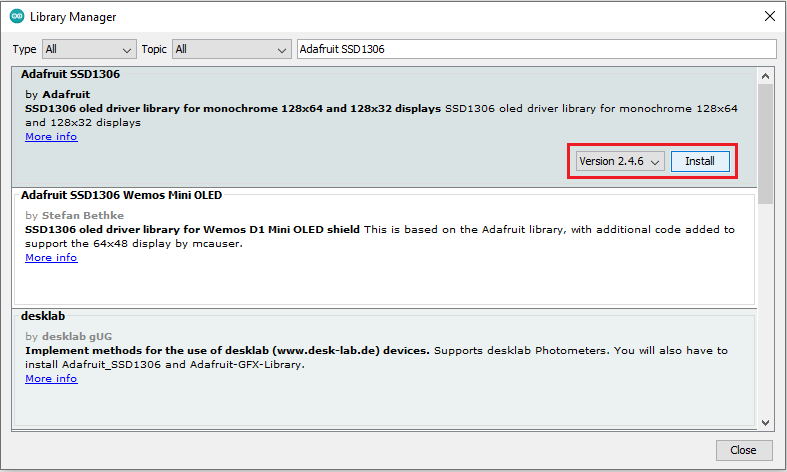
A popup will open. It will ask to install the missing dependencies – Adafruit GFX Library. Select Install all, it will install both Adafruit SSD1306 and Adafruit GFX Library.
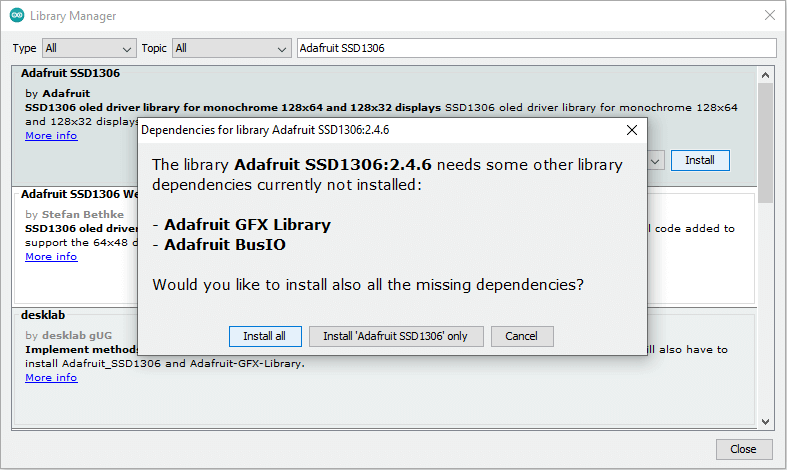
By default, the Adafruit SSD1306 library is configured for a 128×32 pixel display. To make it compatible with a 128×64 pixel display you need to make small changes to the Adafruit_SSD1306.h file.
Find the Adafruit_SSD1306.h file in the Arduino Library folder. Generally, it is located at Documents\Arduino\libraries on windows systems. There you will find the Adafruit_SSD1306.h file inside the Adafruit_SSD1306 folder.
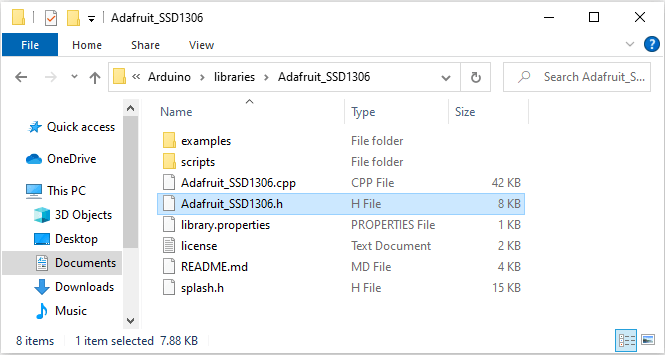
Open the file in a text editor and find the line where the display is defined.
#define SSD1306_128_64
Uncomment the #define SSD1306_128_64
and comment out the #define SSD1306_128_32
.
After editing it should look like this –
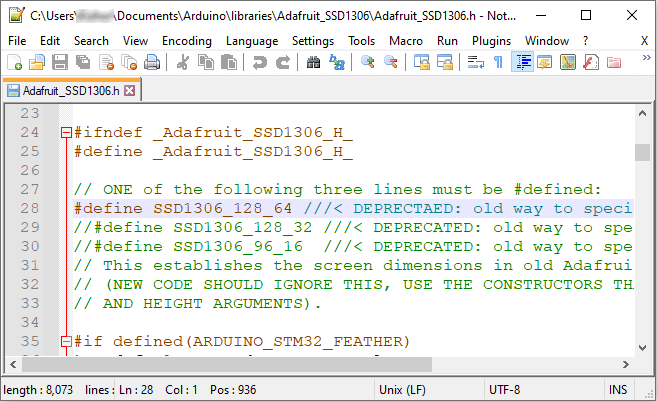
Now, save the file and restart the Arduino IDE to load the libraries properly.
Common Functions and Their Parameters
Before driving into programming let’s take a look at some common functions and their parameters.
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
This will define a display object with screen width, screen height, and a reset pin for the display. &Wire is used for the I2C communication protocol. OLED_RESET is for the reset pin. If you have a separate reset pin on the OLED connect that pin to an Arduino pin and put that pin number in the place of OLED_RESET
. or you can use -1 to use the Arduino reset pin to reset the display.
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
This will initialize the display with the I2C address 0x3C
, and SSD1306_SWITCHCAPVCC
will generate a display voltage of 3.3V internally.
display.clearDisplay()
– Clears the display buffer.display.display()
– will print on the screen from available data on the display ram. You MUST call display()
function after print()
or draw to make them visible on screen!display.setCursor()
– Sets the cursor at positions x, y.display.setTextSize()
– Sets the size of the text.display.print()
– Prints letter or number.display.drawPixel(x,y, color)
– plot a pixel in the x,y coordinates.
Testing the OLED
After wiring the OLED display with the Arduino and installing the necessary library you need to check if everything is working perfectly.
Upload the below code to your Arduino board. It will fill all the pixels of your OLED line by line. If any pixel was damaged you can identify it.
#include <SPI.h>
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin)
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
void setup() {
display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // initialize with the I2C addr 0x3C
display.clearDisplay(); // Clear the buffer
fillDisplay(); // Fills the display line by line
}
void loop() {
}
void fillDisplay(void) {
display.clearDisplay();
for (int j = 0; j < 63; j++) {
for (int i = 0; i < 127; i++) {
display.drawPixel(i, j, WHITE);
}
display.display();
delay(2);
}
}
You can also use one example from the library to test and understand various functions available in the Adafruit SSD1306 library.
You can find this example under Arduino IDE’s example section. Go to File > Examples > Adafruit SSD1306 and select the example for your display.
Printing Text & Numbers
The Adafruit SSD1306 library has several functions to print text and numbers to the OLED display. In this section, you will learn how to print text and numbers in the display.
First, I will give an example then I will explain how the code works.
Arduino OLED Code to Print Text and Numbers
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
void setup() {
// initialize with the I2C addr 0x3C
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
// Clear the buffer.
display.clearDisplay();
// Display Text
display.setTextSize(3);
display.setTextColor(WHITE);
display.setCursor(0, 0);
display.print("Hello!");
display.display();
}
void loop() {}
Upload the code to the Arduino board and your display will look like this:-
Explaining the code
First, you need to import the necessary library. The Wire library is used for the I2C communication protocol. If you use an SPI OLED display use the SPI library.
Adafruit_SSD1306 library is used to control SSD1306 OLED displays and Adafruit_GFX library is used for graphical functionality like drawing points, lines, circles, etc.
Though you don’t need Adafruit GFX library for this particular code but it will be required later.
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
Then you need to define OLED height and width. Here we are using a 128×64 pixel so we set the width 128 and height 64. In case you use different size OLEDs change the value accordingly.
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
Then we create a display object with a previously defined width and height. &Wire
is used because we are using the I2C communication protocol. The last parameter is for the reset pin. If your display has a separate reset pin connect that pin to the Arduino and use that pin number here. Otherwise use -1 to use the Arduino reset pin to reset the display.
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
In the setup section, we initialize the display with begin()
methode. SSD1306_SWITCHCAPVCC
is used to generate display voltage from 3.3V internally and 0x3C
is the I2C address of the display.
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
After initializing the display we use clearDisplay()
function to clear the display buffer.
display.clearDisplay();
Set the size of the text using the setTextSize()
function. You can set any size starting from 1 but use a suitable size for your text to display properly on the screen. We will use text size 3.
Then set the text color using the setTextColor()
function. WHITE makes the text bright and the background dark.
display.setTextSize(3);
display.setTextColor(WHITE);
Before printing anything we need to set the cursor position using setCursor(x,y)
function. Increasing the x value will move the cursor to the right and increasing the y value will move the cursor to the bottom.
print()
will send any text, character, or number to the Display Data RAM (GDDRAM)
display.setCursor(0, 0);
display.print("Hello!");
display()
will print what is on the Display Data RAM (GDDRAM) or buffer. So a print()
function alone can’t display data to the screen. You need to use the display()
function after print()
to see the actual data on the screen.
display.display();
Draw Different Shapes (Circle, Rectangle, Triangle, etc)
In this section, you will learn how to draw different shapes on the screen. Adafruit GFX library has several functions to draw common shapes easily on the screen.
First, we will discuss different functions available on the Adafruit GFX library to draw different shapes then we will go to the complete coding.
Draw Pixel
You can draw a pixel on the display by calling the drawPixel()
function. It requires three parameters. An X, Y coordinate, and a color.
If you want to draw a pixel at 10,10 positions on the display, you can do it like this –
display.clearDisplay();
display.drawPixel(10, 10, WHITE);
display.display();
Drawing Lines
You can draw a line using the drawLine()
function. It requires the coordinates of the starting point and endpoint of the line and the color of the line.
The below code will draw a line from 0, 0 positions to 127, 63 positions i.e. upper left corner to lower right corner.
display.clearDisplay();
display.drawLine(0, 0, 127, 63, WHITE);
display.display();
Draw a Rectangle
You can draw a rectangle by calling the drawRect()
function. It requires the starting coordinate (from where they will start), the height and width of the rectangle in pixels, and the color of the rectangle.
The below code will draw a rectangle from 0, 0 coordinates, and the height and width would be 64 and 32 pixels respectively.
display.clearDisplay();
display.drawRect(0, 0, 64, 32, WHITE);
display.display();
drawRect()
will only draw the outline of the rectangle. You can fill the entire area by using the fillRect()
function. It requires the same parameter as drawRect()
.
display.fillRect(0, 0, 64, 32, WHITE);
Draw Circles
You can draw a circle using the drawCircle()
function. It requires a coordinate for the center of the circle, a radius in pixels, and a color.
The below code will draw a circle with a 10-pixel radius at (31, 15) position.
display.clearDisplay();
display.drawCircle(31, 15, 10, WHITE);
display.display();
You can fill the circle using the fillCircle()
function. It requires the same parameter as drawCircle()
.
display.fillCircle(64, 32, 31, WHITE);
Rounded Rectangle
You can draw a rectangle with a rounded corner using drawRoundRect()
function. It requires the same parameters as drawRect()
with an additional parameter to increase or decrease the radius of the corner rounding.
The below code will draw a 64×32 pixel rounded rectangle from the (0, 0) position with 8-pixel corner rounding.
display.clearDisplay();
display.drawRoundRect(0, 0, 64, 32, 8, WHITE);
display.display();
Similarly, you can fill a rounded rectangle using fillRoundRect()
.
display.fillRoundRect(0, 0, 64, 32, 8, INVERSE)
;
Triangle
You can draw a triangle using the drawTriangle()
function. It requires the three coordinates of the triangle and the color of the triangle.
The below code will draw a triangle by connecting (63, 0), (31, 63), (95, 63) – these three dots.
display.clearDisplay();
display.drawTriangle(63, 0, 31, 63, 95, 63, WHITE);
display.display();
You can fill the triangle using fillTriangle()
function.
display.fillTriangle(0, 0, 0, 32, 64, 32, WHITE);
OLED Code to Draw Different Shapes
The below code will draw different shapes on the OLED for 2 second time duration.
#include <SPI.h>
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
// Declaration for an SSD1306 display connected to I2C (SDA, SCL pins)
#define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin)
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
#define delay_short 400
#define delay_long 2000
void setup() {
// initialize with the I2C addr 0x3C
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
// Clear the buffer
display.clearDisplay();
display.drawPixel(31, 15, WHITE);
display.display();
delay(delay_short);
display.drawPixel(95, 15, WHITE);
display.display();
delay(delay_short);
display.drawPixel(95, 47, WHITE);
display.display();
delay(delay_short);
display.drawPixel(31, 47, WHITE);
display.display();
delay(delay_short);
display.drawPixel(63, 31, WHITE);
display.display();
delay(delay_long);
display.clearDisplay();
display.drawLine(0, 0, 127, 63, WHITE);
display.display();
delay(delay_short);
display.drawLine(0, 63, 127, 0, WHITE);
display.display();
delay(delay_short);
display.drawLine(63, 0, 63, 63, WHITE);
display.display();
delay(delay_short);
display.drawLine(0, 31, 127, 31, WHITE);
display.display();
delay(delay_long);
display.clearDisplay();
display.drawRect(0, 0, 63, 31, WHITE);
display.display();
delay(delay_short);
display.drawRect(31, 15, 63, 31, WHITE);
display.display();
delay(delay_short);
display.drawRect(63, 31, 63, 31, WHITE);
display.display();
delay(delay_long);
display.clearDisplay();
display.fillRect(0, 0, 42, 21, WHITE);
display.display();
delay(delay_short);
display.fillRect(42, 21, 42, 21, WHITE);
display.display();
delay(delay_short);
display.fillRect(84, 42, 42, 21, WHITE);
display.display();
delay(delay_long);
display.clearDisplay();
display.drawRoundRect(0, 0, 64, 32, 8, WHITE);
display.display();
delay(delay_short);
display.drawRoundRect(64, 32, 64, 32, 16, WHITE);
display.display();
delay(delay_long);
display.clearDisplay();
display.fillRoundRect(0, 0, 64, 32, 8, WHITE);
display.display();
delay(delay_short);
display.fillRoundRect(64, 32, 64, 32, 16, WHITE);
display.display();
delay(delay_long);
display.clearDisplay();
display.drawCircle(31, 15, 10, WHITE);
display.display();
delay(delay_short);
display.drawCircle(95, 47, 10, WHITE);
display.display();
delay(delay_short);
display.drawCircle(95, 15, 10, WHITE);
display.display();
delay(delay_short);
display.drawCircle(31, 47, 10, WHITE);
display.display();
delay(delay_short);
display.drawCircle(64, 32, 10, WHITE);
display.display();
delay(delay_long);
display.clearDisplay();
display.fillCircle(31, 15, 10, WHITE);
display.display();
delay(delay_short);
display.fillCircle(95, 47, 10, WHITE);
display.display();
delay(delay_short);
display.fillCircle(95, 15, 10, WHITE);
display.display();
delay(delay_short);
display.fillCircle(31, 47, 10, WHITE);
display.display();
delay(delay_short);
display.fillCircle(64, 32, 10, WHITE);
display.display();
delay(delay_long);
display.clearDisplay();
display.drawTriangle(63, 0, 31, 63, 95, 63, WHITE);
display.display();
delay(delay_short);
display.drawTriangle(0, 0, 0, 63, 31, 0, WHITE);
display.display();
delay(delay_short);
display.drawTriangle(127, 0, 127, 63, 95, 0, WHITE);
display.display();
delay(delay_long);
display.clearDisplay();
display.fillTriangle(63, 0, 31, 63, 95, 63, WHITE);
display.display();
delay(delay_short);
display.fillTriangle(0, 0, 0, 63, 31, 0, WHITE);
display.display();
delay(delay_short);
display.fillTriangle(127, 0, 127, 63, 95, 0, WHITE);
display.display();
delay(delay_long);
}
void loop() {
}
Invert The Display
The library has an interesting function that can invert the display – invertDisplay()
. Applying this function will change the bright area to dark and brighten the dark area. It will work with any text and graphics.
You can pass true or 1 as an argument to invert the display and false or 0 to reverse it back to the previous position.
Here we will first draw a filled circle then invert the display and reverse it back to see how these functions work.
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin)
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
#define delay_short 400
#define delay_long 1000
#define delay_ms 2000
void setup() {
// initialize with the I2C addr 0x3C
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
// Clear the buffer
display.clearDisplay();
// Draw circle
display.clearDisplay();
display.fillCircle(63, 31, 30, WHITE);
display.display();
delay(delay_short);
display.invertDisplay(true);
delay(1000);
display.invertDisplay(false);
delay(1000);
}
void loop() {
}
Inverted Text and Numbers
We can invert text and numbers using the setTextColor()
function. This function needs two parameters – a font color and a background color.
To set the background bright and the text/number dark use the function like this.
display.setTextColor(BLACK, WHITE);
Arduino OLED Code to Print Inverted Text and Numbers
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin)
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
void setup() {
// initialize with the I2C addr 0x3C
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
// Display Inverted Text
display.clearDisplay();
display.setTextSize(3);
display.setTextColor(BLACK, WHITE); // 'inverted' text
display.setCursor(0, 20);
display.println("Hello!");
display.display();
delay(2000);
}
void loop() {
}
This function will work on both texts and numbers.
Using Different Fonts from The Library
Arduino OLED Scrolling Text
Adafruit’s SSD1306 library has a set of libraries to scroll text on the display.
startscrollright()
– Scroll pages from left to right.startscrollleft()
– Scroll pages from right to left.startscrolldiagright()
– Scroll pages from the left bottom corner to the upper right corner.startscrolldiagleft()
– Scroll pages from the right bottom corner to the upper left corner.stopscroll()
– Stops scrolling.
All these functions except stopscroll()
needs two parameters, start page, and end page. As you learned in the OLED memory mapping section there are seven pages in SSD1306’s RAM. you can move all eight pages or a group of pages or a single page.
startscrollright(0, 0)
will only move what is on the first page of the display to the right.startscrollright(2, 5)
will move the middle selection of the display. Page number 2,3,4, and 5. And startscrollright(0, 7)
will move the whole screen.
Note:- all these functions work with both decimal and hexadecimal values. So startscrollright(0, 7)
and startscrollright(0x00, 0x07)
will give you the same results.
The below example will implement those functions.
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin)
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
void setup() {
// initialize with the I2C addr 0x3C
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
display.clearDisplay();
display.setTextSize(1);
display.setTextColor(WHITE);
display.println("Scrolling");
display.println("Text");
display.display();
display.startscrollright(0, 0);
delay(3000);
display.stopscroll();
display.startscrollleft(0, 0);
delay(3000);
display.stopscroll();
display.startscrollright(1, 1);
delay(3000);
display.stopscroll();
display.startscrollleft(1, 1);
delay(3000);
display.stopscroll();
display.clearDisplay();
display.setTextSize(1);
display.setTextColor(WHITE);
display.println("Scrolling");
display.println("Display");
display.display();
display.startscrolldiagright(0, 7);
delay(5000);
display.stopscroll();
display.startscrolldiagleft(0, 7);
delay(5000);
display.stopscroll();
}
void loop() {
}
Draw ASCII Characters
In this section, you will learn how to draw ASCII characters and symbols for the OLED display. While the print()
and println()
function prints human-readable ASCII text the write()
function prints ASCII characters and symbols on the display.
The write()
function needs a decimal number as an argument. Every ASCII character is assigned a decimal number to recognize it. You need this number as the argument of this function.
You can find those numbers on Code page 437. For example, if you want to print a smile on the display you can print it using –
display.write(2);
The below code will print various ASCII characters on the display.
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin)
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
void setup() {
// initialize with the I2C addr 0x3C
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
display.clearDisplay();
display.setTextSize(2);
display.setTextColor(WHITE);
display.write(2);
display.setCursor(20, 0);
display.write(3);
display.setCursor(40, 0);
display.write(14);
display.setCursor(60, 0);
display.write(38);
display.setCursor(80, 0);
display.write(42);
display.display();
}
void loop() {
}
Display Custom Graphics (Bitmap Image)
You can draw a bitmap image on the display using the drawBitmap()
function. To use this function you need to create the bitmap array of the image first. There are various online and offline tools available for that. Here we will use image2cpp to create a bitmap array of the below image.
Download the image and upload it to image2cpp.
In the settings page change the canvas size to 128×64. Also change the scaling from the original size to scale to fit, keeping proportions.
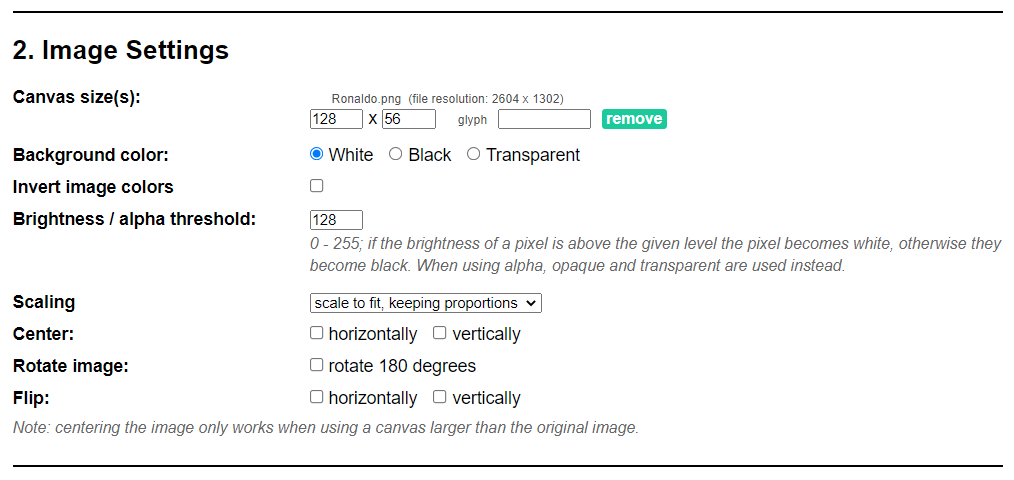
In the preview section, you can see what your output looks like.
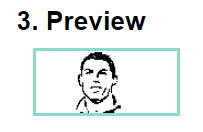
In the output section, you could select plain bytes or Arduino code. Then select generate code.
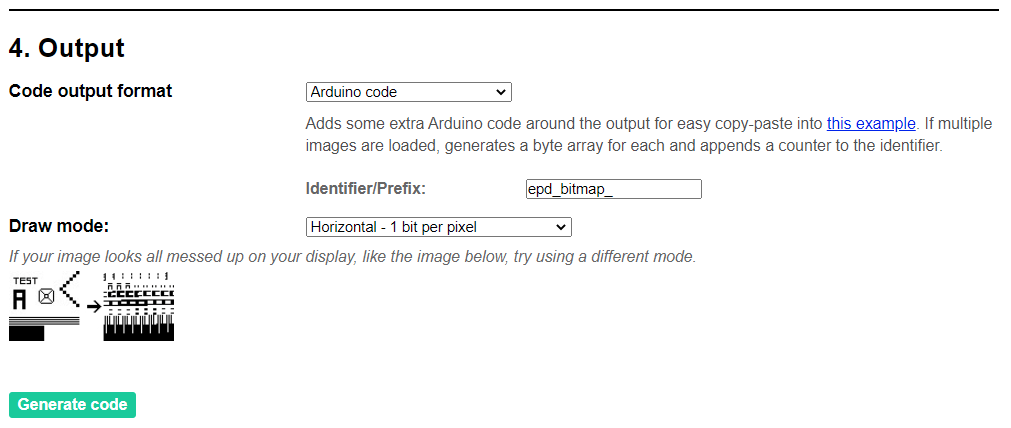
You will find the bitmap array for this image in the code box. We will use that array to display that image on the screen.
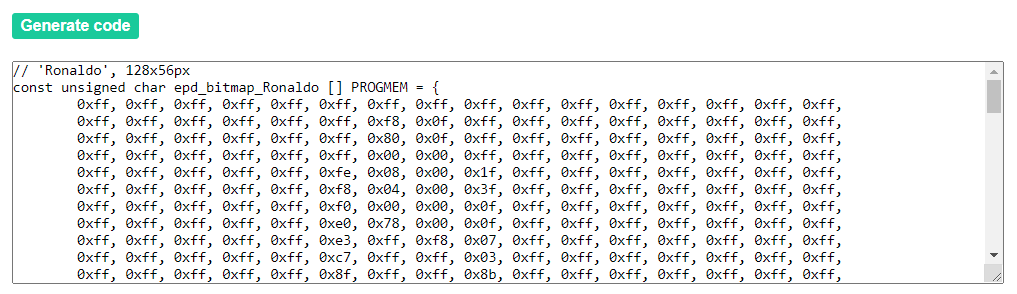
In the coding section, we first store that array in the flash memory using
const unsigned char ronaldo [] PROGMEM = {}
and print this using display.drawBitmap(0, 0, ronaldo, 128, 64, WHITE);
where 0, 0
is used to print the bitmap image from 0, 0 location, ronaldo
is the name of the bitmap array, and 128, 64
is used for a 128×64 display.
The final code will look like this –
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin)
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
// Bitmap of ronaldo Image
const unsigned char ronaldo [] PROGMEM = {
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf8, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x0f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf8, 0x18, 0x00, 0x0f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe0, 0x00, 0x00, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xc0, 0x00, 0x00, 0x03, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0xf8, 0x00, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x87, 0xff, 0xe0, 0x01, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x0f, 0xff, 0xff, 0x00, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x1f, 0xff, 0xff, 0xc2, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x1f, 0xff, 0xff, 0xe0, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x3f, 0xff, 0xff, 0xf0, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x3f, 0xff, 0xff, 0xe0, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0x7f, 0xff, 0xff, 0xf0, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0x7f, 0xff, 0xff, 0xf0, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0x7f, 0xff, 0xff, 0xf0, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0x79, 0xff, 0xff, 0xf0, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0xe0, 0x3f, 0xc0, 0x38, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0xfe, 0x0f, 0x83, 0xbc, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0xfc, 0x0f, 0x87, 0xfc, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0xf0, 0x1f, 0x00, 0xfe, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0xf6, 0xff, 0x10, 0x3c, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xf0, 0xff, 0xfe, 0x7f, 0x7e, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xf6, 0xff, 0xfe, 0x7f, 0xfe, 0x0f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xe2, 0xff, 0xfe, 0x7f, 0xfe, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xe7, 0xff, 0xff, 0x7f, 0xfe, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xe7, 0xff, 0xff, 0xff, 0xfe, 0x77, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xe7, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xe7, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xff, 0xe0, 0x7f, 0xff, 0xef, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xf7, 0x7f, 0xf9, 0x3f, 0xff, 0xef, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xf3, 0x7f, 0xff, 0xff, 0xfe, 0xdf, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfb, 0x7f, 0xff, 0xff, 0xfc, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0x7f, 0xff, 0xff, 0xfc, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x7f, 0xe0, 0x7f, 0xfc, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x7f, 0x8f, 0x0f, 0x7d, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x7f, 0x3f, 0x8e, 0x7d, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x7f, 0xff, 0xfe, 0x65, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x3f, 0xff, 0xfe, 0xed, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x3f, 0xff, 0x7c, 0xed, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xbf, 0xf0, 0xfd, 0xed, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x9f, 0xfb, 0xfb, 0xed, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xcf, 0xff, 0xf3, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xef, 0xff, 0xf7, 0xde, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xe7, 0xff, 0xff, 0x9e, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x9b, 0xff, 0xfe, 0x3e, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x1c, 0xff, 0xfc, 0x7e, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x7e, 0x7e, 0x70, 0xfe, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0xef, 0x00, 0x01, 0xff, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfd, 0xcf, 0x80, 0x03, 0xff, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xf9, 0xcf, 0xc0, 0x07, 0xff, 0x0f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xf3, 0xcf, 0xe0, 0x1f, 0xff, 0x0f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xf7, 0xcf, 0xf8, 0xff, 0xff, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xe7, 0xcf, 0xff, 0xff, 0xff, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xef, 0xe7, 0xff, 0xff, 0xff, 0xf8, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xcf, 0xe7, 0xff, 0xff, 0xff, 0xe0, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xdf, 0xf7, 0xff, 0xff, 0xff, 0xc0, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0x9f, 0xff, 0xff, 0xbf, 0xff, 0x81, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfb, 0xff, 0xff, 0xfc, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfb, 0xff, 0xff, 0xe0, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xf3, 0xfd, 0xfc, 0xff, 0x00, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xfc, 0x7d, 0xff, 0xfc, 0x00, 0x7d, 0xff, 0xff, 0xff, 0xff, 0xff
};
void setup() {
// initialize with the I2C addr 0x3C
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
// Clear the buffer.
display.clearDisplay();
// Display bitmap
display.drawBitmap(0, 0, ronaldo, 128, 64, WHITE);
display.display();
}
void loop() {
}
Conclusions
I hope you learn the basics of OLED display and how it works with Arduino. Now you can easily add an OLED display to your Arduino projects.
Use the comment section below to share your projects and ideas. Also, subscribe to our newsletter for regular project updates.