The thermistor is basically a thermal resistor. That means its resistance changes according to the change in temperature of the environment. We can use a thermistor to measure the temperature of the environment.
If you want simple, inexpensive, and accurate components to get the temperature data for your project thermistor would be a good choice. A thermistor would be ideal for your remote weather stations project, home automation systems, temperature control, protection circuit, etc.
In this tutorial, I will explain how a thermistor works and how you can build a small circuit with Arduino and a thermistor that displays the temperature on the serial monitor or on an LCD display.
Table of Contents
How Thermistor Works
A thermistor is a variable resistor whose resistance changes with the temperature. Change in resistance means a change in voltages. We can use an Arduino analog pin to measure that voltage changes and from that, we can calculate the temperature value.
There are basically two types of thermistors – Negative Temperature Coefficient (NTC) thermistors, and Positive Temperature Coefficient (PTC) thermistors. In negative Temperature Coefficient (NTC) thermistors, resistance decreases as temperature increases. In Positive Temperature Coefficient (PTC) thermistors, resistance increases as temperature increases.
NTC is the most used thermistor and in this tutorial, I will use an NTC thermistor.
Basic Thermistor Circuit
If you want to use the thermistor with an MCU (Arduino) you will need a voltage divider circuit configuration like below. The value of the resistor should be nearly equal to the resistance of your thermistor. I am using a 100K Ω NTC thermistor and a 100K Ω resistor.
Connect the known resistor in series with the thermistor like below. Then connect another end of the thermistor to +5v and the remaining end of the known resistor to the ground. Now you can take the output from the middle of the thermistor and 100K Ω resistor.
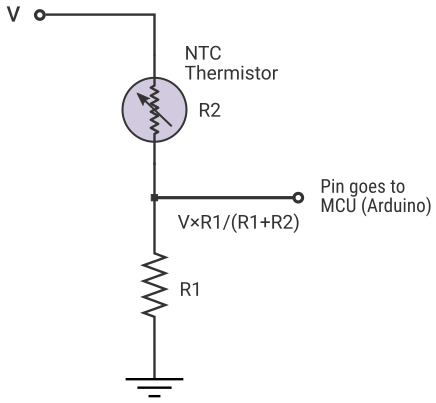
Connect a Thermistor with The Arduino
Follow the below circuit diagram to connect a thermistor with the Arduino. Here you can see that one leg of the thermistor is connected to the +5v pin on the Arduino and another leg is connected to the Analog pin A3. The same leg which is connected to the analog pin is connected to the ground through a 100K Ω resistor.
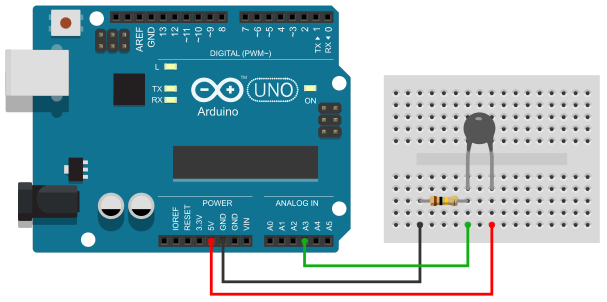
Calculating Temperature
Arduino ADC (Analog Pin) only reads the voltage levels. You need to do some calculations to get the temperature value from that data. There are various formulas to calculate temperature values from the resistance of the Thermistor. Here I will discuss the two most effective formulas to calculate the temperature value.
The first one is the Steinhart–Hart equation.

Where,
T is the temperature in Kelvins.
R is the resistance of the thermistor at T (in Ohms).
A, B and C are the Steinhart–Hart coefficients.
To solve this equation you need to know the value of the three constants – A, B, and C. These constants will vary depending on the type and model of the thermistor and the temperature range of interest.
You can find these values on the datasheet. Or you can calculate this manually by measuring the resistance of the thermistor at three different temperatures. From there you will get three equations and you will find the value of A, B, and C by solving these equations.
Another equation is the β parameter equation.
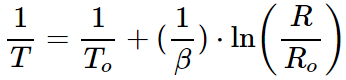
Where,
T is the temperature in Kelvins.
R is the resistance of the thermistor at T (in Ohms).
Ro is the resistance of the thermistor at a reference temperature of To (e.g the resistance at room temperature).
Display Temperature Data on Arduino Serial Monitor
In this section, I will show you how to read the temperature data from an NTC thermistor and display it in the serial monitor of the Arduino.
After connecting a thermistor to the Arduino, upload the below code to the Arduino board. Open the serial monitor and you will get the temperature in Celsius and Fahrenheit.
Arduino Code
This code used the Steinhart–Hart equation to find the temperature value.
int thermistorPin = A3;
int Vo;
float R1 = 10000;
float logR2, R2, tKelvin, tCelsius, tFahrenheit;
float c1 = 1.009249522e-03, c2 = 2.378405444e-04, c3 = 2.019202697e-07;
void setup() {
Serial.begin(9600);
}
void loop() {
Vo = analogRead(thermistorPin);
R2 = R1 * (1023.0 / (float)Vo - 1.0); // resistance of the Thermistor
logR2 = log(R2);
tKelvin = (1.0 / (c1 + c2 * logR2 + c3 * logR2 * logR2 * logR2));
tCelsius = tKelvin - 273.15;
tFahrenheit = (tCelsius * 9.0) / 5.0 + 32.0;
Serial.print("Temperature: ");
Serial.print(tFahrenheit);
Serial.print(" F; ");
Serial.print(tCelsius);
Serial.println(" C");
delay(500);
}
Explaining the Code
Here you can see that I define some variables and constants that I will use later in the program. thermistorPin is used to hold the Arduino pin number connected to the thermistor. Vo is used to holding the analog read input. R1 is the resistance of the known resistor (in ohms) and R2 is the resistance of the thermistor. tKelvin, tCelsius, tFahrenheit will hold the temperature data in Kelvin, Celsius, and Fahrenheit respectively. c1, c2, and c3 are the Steinhart–Hart coefficients.
In the setup section, I start the serial communication at 9600 baud. Now coming to the loop section, you can see that first I read the analog pin, and from that, I calculate the current resistance of the thermistor. Then I calculate the value of the log(R2) and in the next line, I calculate the temperature value in kelvin using the Steinhart–Hart equation.
The rest of the program is very easy. you can easily convert the Kelvin to Celcius and Celcius to Fahrenheit. Now you can use Arduino’s serial print function to print the temperature values to the Arduino serial monitor.
Code for the β parameter equation
The below code used the β parameter equation to find the temperature value.
int thermistorPin = A3;
int Vo; // Holds the ADC Value
float R2, tKelvin, tCelsius, tFahrenheit;
const float Beta = 3974.0;
const float roomTemp = 298.15; // room temperature in Kelvin
const float Ro = 10000.0; // Resistance of the thermistor at roomTemp
const float R1 = 9710.0; // Resistnce of the known resistor
void setup() {
Serial.begin(9600);
}
void loop() {
Vo = analogRead(thermistorPin);
R2 = R1 * (1023.0 / (float)Vo - 1.0); // Resistance of the Thermistor
tKelvin = (Beta * roomTemp) /
(Beta + (roomTemp * log(R2 / Ro)));
tCelsius = tKelvin - 273.15;
tFahrenheit = (tCelsius * 9.0) / 5.0 + 32.0;
Serial.print("Temperature: ");
Serial.print(tFahrenheit);
Serial.print(" F; ");
Serial.print(tCelsius);
Serial.println(" C");
delay(500);
}
Display Thermistor Temperature Data on LCD
In this section, I will show you how to display the temperature data on an LCD. If you are not familiar with LCD displays read the below articles :
For this tutorial, I will use an I2C LCD because it requires less connection and waring. But you can easily connect a normal LCD display using the above tutorial.
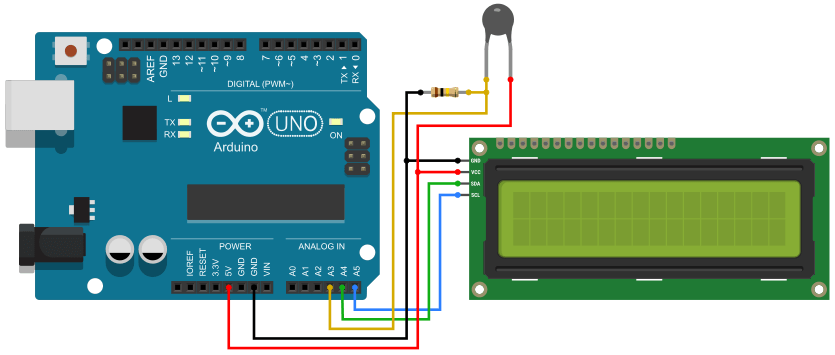
Arduino Code
Here you need to install the Arduino LiquidCrystal I2C Library to work with the I2C LCD. So first install this library and then upload the below code to the Arduino board.
#include <dht.h>
#include <LiquidCrystal_I2C.h>
int thermistorPin = A3;
int Vo; // Holds the ADC Value
const float Beta = 3974.0;
const float roomTemp = 298.15; // room temperature in Kelvin
const float Ro = 10000.0; // Resistance of the thermistor at roomTemp
const float R1 = 9710.0; // Resistnce of the known resistor
float R2, tKelvin, tCelsius, tFahrenheit;
LiquidCrystal_I2C lcd(0x27, 16, 2);
void setup() {
lcd.init();
lcd.clear();
lcd.backlight(); // Make sure backlight is on
}
void loop() {
Vo = analogRead(thermistorPin);
R2 = R1 * (1023.0 / (float)Vo - 1.0); // Resistance of the Thermistor
tKelvin = (Beta * roomTemp) /
(Beta + (roomTemp * log(R2 / Ro)));
tCelsius = tKelvin - 273.15;
tFahrenheit = (tCelsius * 9.0) / 5.0 + 32.0;
lcd.setCursor(0, 0);
lcd.print("Temp: ");
lcd.print(tFahrenheit);
lcd.print((char)223);
lcd.print("F");
delay(500);
}