The Raspberry Pi Pico is the latest addition to the Raspberry Pi family. But unlike other pi boards, it is not a Linux computer. It is a new microcontroller board with a powerful new chip the RP2040. RP2040 is a dual-core Arm Cortex-M0+ processor with 264KB internal RAM and support for up to 16MB of off-chip Flash.
In this tutorial, you will learn how to connect a Raspberry Pi Pico to your computer. How to program it using Micropython as well as using C and C++. And at the end, we will give some examples like, how to blink the onboard LED and external led, how to use the PWM to control the brightness of a led, and how to read the analog sensor data.
Table of Contents
- 1 The RP2040
- 2 RP2040 Specifications
- 3 Raspberry Pi Pico Features
- 4 Raspberry Pi Pico Peripherals
- 5 Raspberry Pi Pico GPIO Pinout
- 6 MicroPython on Raspberry Pi Pico
- 7 Start Coding
- 8 Raspberry Pi Pico onboard LED Blinking
- 9 Blink an External LED
- 10 Working with PWM
- 11 Analog Input in Raspberry Pi Pico
- 12 Control the brightness of an LED
- 13 Working With Push Button
- 14 Conclusion
The RP2040
Raspberry Pi Pico is built around RP2040, the new microcontroller by Raspberry Pi. It is designed to work with Micropython and C/C++. Highly flexible design and wide range of I/O make it suitable for a wide range of microcontroller applications. Its ease of use and low price makes it popular among the maker’s community.
RP2040 Specifications
- Dual ARM Cortex-M0+ @ 133MHz
- 264kB on-chip SRAM in six independent banks
- Support for up to 16MB of off-chip Flash memory via dedicated QSPI bus
- DMA controller
- Interpolator and integer divider peripherals
- On-chip programmable LDO to generate core voltage
- 2 on-chip PLLs to generate USB and core clocks
- 30 GPIO pins, 4 of which can be used as analog inputs
Raspberry Pi Pico Features
RP2040 microcontroller
Dual Cortex M0+ processors, up to 133 MHz
264 kB of embedded SRAM
2MB of On-board Flash Memory
Two Programmable I/O Block (PIO)
Micro-USB B Port for Power, Data, and programming the Flash memory.
3-pin ARM Serial Wire Debug (SWD) port
Raspberry Pi Pico Peripherals
- 30 multi-function General Purpose IO (4 can be used for ADC)
- 12-bit 500ksps Analogue to Digital Converter (ADC)
- Various digital peripherals
- 2 × UART, 2 × I2C, 2 × SPI, 16 × PWM channels
- 1 × Timer with 4 alarms, 1 × Real Time Counter
- 2 × Programmable IO (PIO) blocks, 8 state machines total
- Flexible, user-programmable high-speed IO
- Can emulate interfaces such as SD Card and VGA
Raspberry Pi Pico GPIO Pinout
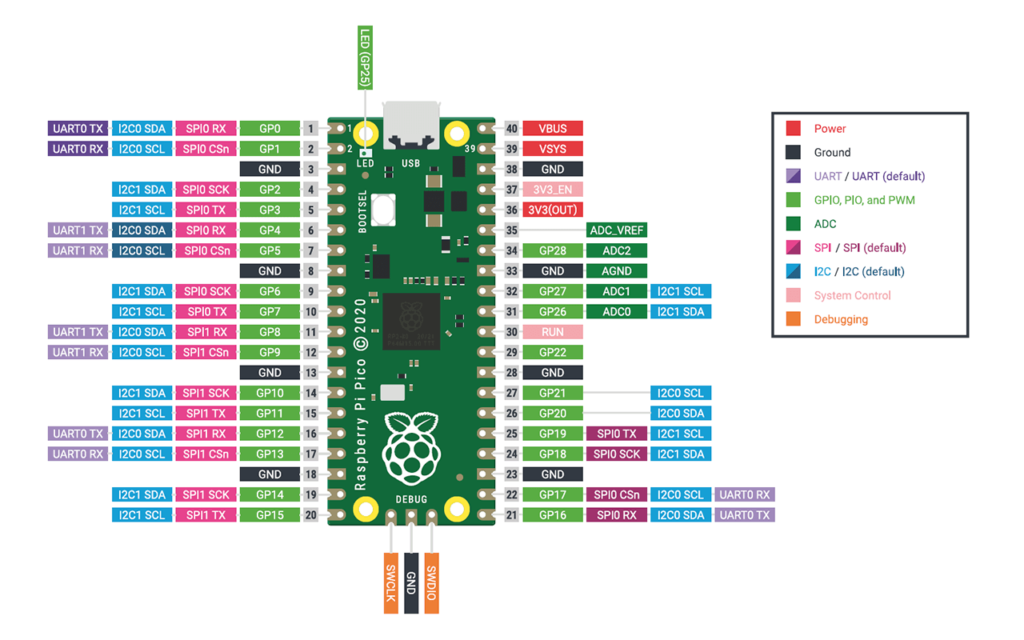
MicroPython on Raspberry Pi Pico
Micropython is a version of Python 3 optimized to run on a microcontroller. It was created by Damien George in 2014 and was first used with the PyBoard development board. Since then it gained popularity day by day and many forks were created which support a variety of systems and hardware platforms. Writing Micropython code for Raspberry Pi Pico is possible using thonny.
Install Thonny
Thonny is a simple python IDE that also supports MicroPython. It has a built-in interpreter for MicroPython for Raspberry Pi Pico. So you can easily run and upload Micropython code to a Raspberry Pi Pico using thonny. It is available for all three major platforms Windows, Mac, and Linux. Download the IDE from thonny.org and install it on your device.
Open the application and it will look like this :
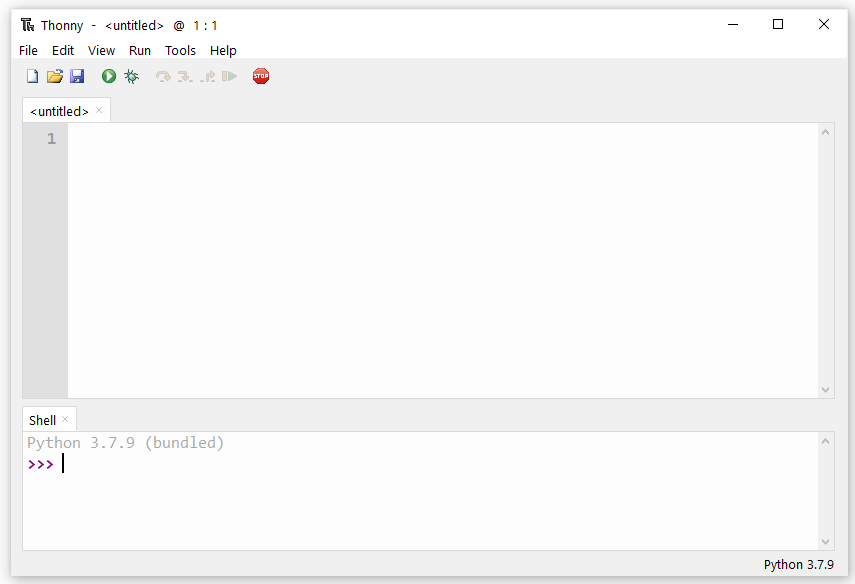
You can use thonny to write standard python code as well as Micropython. To use Micropython code you need to install the firmware first.
Install the MicroPython firmware for Raspberry Pi Pico
To install Micropython firmware for Raspberry Pi Pico you need to press and hold the BOOTSEL button while connecting the Pico to the computer. It will open up the Pico as a mass storage device mode. Then in the bottom right corner of the thonny you can see the python version number.
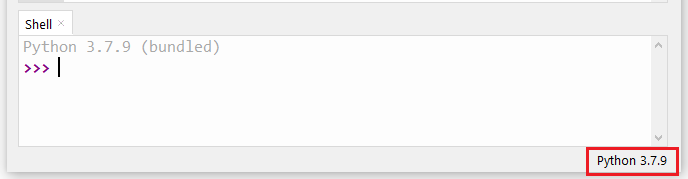
Click here and you will see options like this
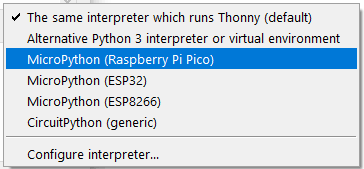
Select the MicroPython (Raspberry Pi Pico) and a dialog box will open up to install the latest firmware to your Raspberry Pi Pico.
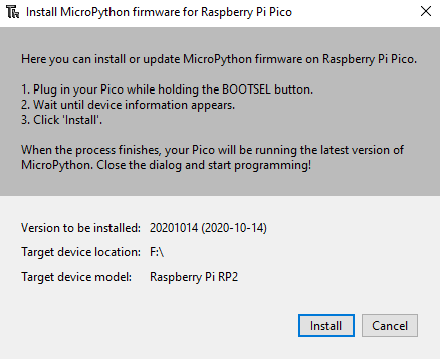
Click the install button to install the firmware and give it some time to complete the installation.
Once you have installed the firmware successfully, you don’t need to install it again. Next time you just connect the Raspberry Pi Pico without pressing the BOOTSEL button and start coding.
Start Coding
You can run Python code on your Raspberry Pi Pico in two ways. Using the main python editor and using the shell below the main code editor.
Shell is very useful for shortcodes or when you try to quickly check the output of a code.
Copy and paste the below code to the shell and hit enter. It will turn on the onboard led.
from machine import Pin
led = Pin(25, Pin.OUT)
led.value(1)
Then simply put led.value(0) this code to the shell and hit enter. It will turn the LED off. From here you can understand that the shell is very useful to run and check small code.
However, when you write a long program using a shell is not very efficient. You can’t edit the code after pressing the enter button on a shell. That’s why you should use the main code editor for a long program.
To use the main code editor enter the below code to the main editor section of the Thonny.
from machine import Pin
led = Pin(25, Pin.OUT)
led.value(1)
Hit the run button and a dialogue box will open. It will give you the option to save the code on This Computer or on the Micropython device. Select Micropython device and save the code with a .py extension. Now you can run the program.
So, you got the basic idea of how you can run a Micropython code on the Raspberry Pi Pico. Now I will give some basic examples.
Raspberry Pi Pico onboard LED Blinking
Raspberry Pi Pico has a green LED beside the USB port. We can control it through coding.
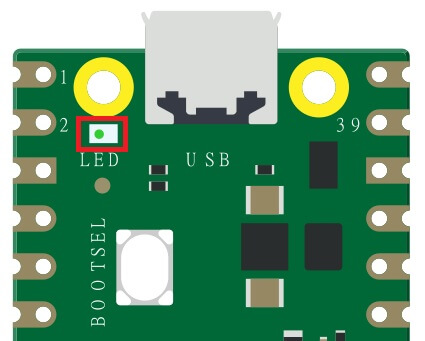
This LED is attached to pin no 25. We need two functions to blink a LED using Mycropython – Pin and Timer. Micropython’s Pin function is used to interact with the GPIO and the Timer function is used to do time-related tasks. So first we need to import the pin and timer from the machine function.
from machine import Pin, Timer
Then we create a LED object named led_pin and attach pin number 25 to it and set that pin as an output pin.
led_pin = Pin(25, Pin.OUT)
We also create a variable tim
for the Timer()
function.
Then we define a function called led_blink
which will toggle the LED when called.
And at the last, we use the timer function to toggle the LED with a periodic time of frequency 1. That means that the LED will blink once within 1 second.
The complete code would look something like this :-
from machine import Pin, Timer
led_pin = Pin(25, Pin.OUT)
tim = Timer()
def blink_led(tim):
led_pin.toggle()
tim.init(freq=1, mode=Timer.PERIODIC, callback=blink_led)
Blink an External LED
Blinking an external LED is pretty simple. You need to make a small circuit on a breadboard for that. Connect the long leg (+ve) of the LED to general pin no 15 with a resistor (~ 220ohm) in series. Connect the short leg (-ve) of the LED to the ground pin of the Raspberry pi Pico.
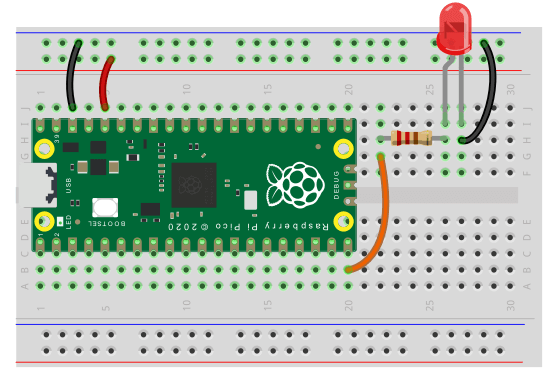
Run the below code and the LED will Start Blinking.
from machine import Pin, Timer
led_pin = Pin(15, Pin.OUT)
tim = Timer()
def blink_led(tim):
led_pin.toggle()
tim.init(freq=1, mode=Timer.PERIODIC, callback=blink_led)
Note :- If you save the file as blink.py
or something like that your code will run until you disconnect the Raspberry Pi Pico from your computer. You have to re-upload the code to the Raspberry Pi Pico when you connect next time.
But if you save your code with the name main.py
it will run automatically every time when you power up your Raspberry Pi Pico.
Working with PWM
Pulse Width Modulation or PWM is a technique to generate analog like signals within a digital system. Raspberry Pi Pico has 16 such pins that can be used to generate PWM signals. We will generate a PWM signal at pin no 15 and use that PWM signal to control the brightness of an LED.
The circuit for this example is the same as the previous circuit. You just have to change the code like below and check the output.
import machine
import utime
pwm_width = 0
led_pin = machine.PWM(machine.Pin(15))
led_pin.freq(1000)
while True:
while pwm_width < 65536:
pwm_width += 100
utime.sleep(0.001)
led_pin.duty_u16(pwm_width)
while pwm_width > 0:
pwm_width -= 100
utime.sleep(0.001)
led_pin.duty_u16(pwm_width)
Explaining the Code
Here you can see that we imported the necessary library first. machine
library is used for pin manipulation and utime
is used for time-related tasks. Then we create a pin object for pin number 15 named led_pin
and set it as a PWM pin. We set the frequency of that pin to 1000 Hz. In the while loop, we gradually increase the value of the variable pwm_width
from 0 to 65536 and pass that value to the led_pin
as PWM width. So the brightness of the LED will increase from 0 to high intensity.
Then we will decrease the value of the variable pwm_width
from 65536 to 0 and use it as the PWM width of led_pin
. So the brightness of the LED will decrease from high intensity to low. Overall it will create a dimming effect.
Analog Input in Raspberry Pi Pico
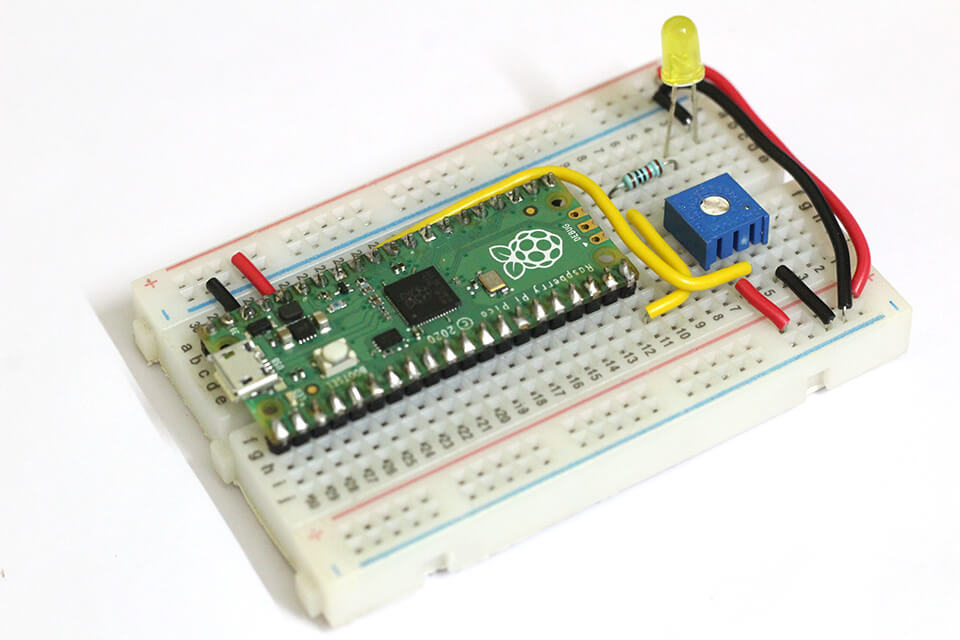
Raspberry Pi Pico has three 12-bit Analog to Digital Converters (ADC) GPIO 26,27,28 (physical pin no – 31, 32, 34). ADC controller uses a 16-bit integer to operate the ADC. That means the analog pin has an input range between 0 to 65535.
In this example, we will use a potentiometer to supply a variable voltage to the ADC. We will read that variable analog voltage and convert it to digital data and print it to the terminal. Then we will use that data to control the brightness of an LED.
So first we will connect a 10k ohm potentiometer with the Pico. For that, we need to connect the potentiometer +ve pin to the 3.3v pin, -ve pin to any ground pin, and middle pin to pin no 26 (ADC0) of the Pico.
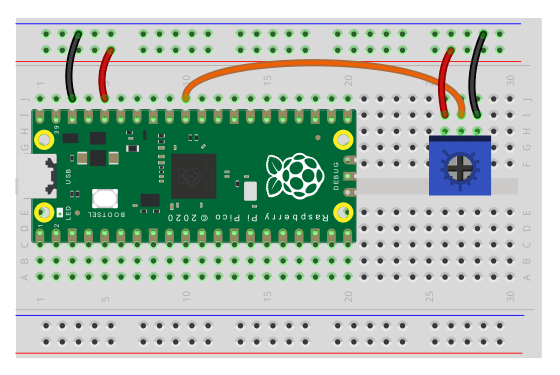
Now upload the below code to your Raspberry Pi Pico and turn the knob. The number on the terminal will increase or decrease as you turn it clockwise or anti-clockwise.
import machine
import utime
pot = machine.ADC(26)
while True:
print(pot.read_u16())
utime.sleep(1)
Explaining the Code
Here you can see that we import the machine
library for GPIO manipulation and the utime
library for time-related tasks. Then we define a variable pot
that will hold the input data.
pot = ADC(Pin(26))
Here “ADC” is used to enable the analog input function of that pin.
In the loop section, we print that data.
print(pot.read_u16())
Here read_u16()
is used to read that data in 16-bit unsigned integer mode. That means input data will vary between 0 to 65535. Then we use a 1-second delay in this continuous loop.
So you have learned how to read analog data using Raspberry Pi Pico. Now we will see how we can use that data to control things.
Control the brightness of an LED
Here in this example, we will control the brightness of an LED using a Potentiometer.
The circuit for this experiment is very simple. You just need to add a LED to the existing circuit. Hope you can add this yourself, otherwise follow the below circuit diagram
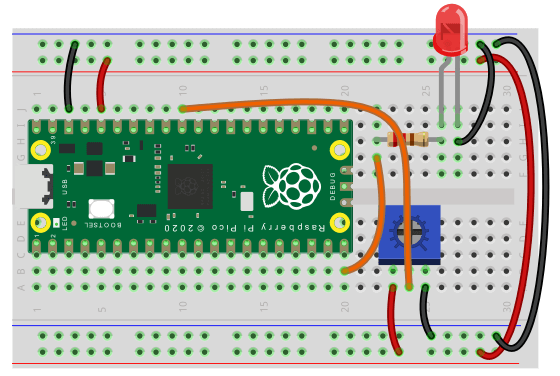
Now upload the below code and turn the knob. The brightness of the LED will increase or decrease as you turn it clockwise or anti-clockwise.
import machine
import utime
led_pin = machine.PWM(machine.Pin(15))
pot = machine.ADC(26)
while True:
led_pin.duty_u16(pot.read_u16())
utime.sleep_ms(2)
Explaining the Code
So here you can see that we read the potentiometer value like before. Then in the while loop, we pass that value to the LED pin as a PWM width so that the brightness of the LED increases with the increasing potentiometer value.
Working With Push Button
Push button is simple yet very useful to make your project more interactive. In this example, we will use a push button to control four different color LEDs.
For this example, you need to make a small circuit like below. We attach a LED to pin number 15 with a series 220-ohm resistor. Then we attach one side of the push button to the 3.3v pin of the Pico and another side to pin number 26.
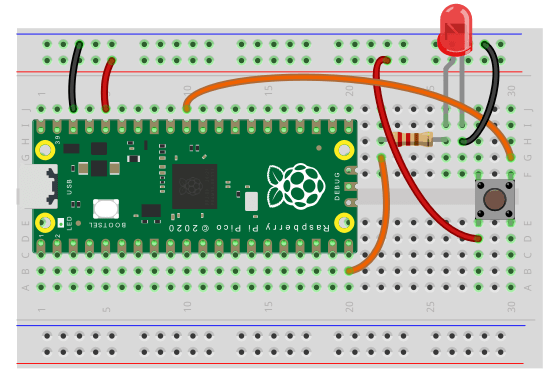
Now upload the below code to your Pico and press the button. The LED will turn on when you press the button once and it will turn off when you press it again.
# BUTTON - Pico - Pin 26 PULL_DOWN
# LED - Pico - Pin 15
from machine import Pin, Timer
import utime
button_pin = Pin(26, Pin.IN, Pin.PULL_DOWN)
led_pin = Pin(15, Pin.OUT)
while True:
if button_pin.value() == 1:
led_pin.toggle()
utime.sleep(0.2)
Explaining the Code
Here you can see that we import the necessary library first. Then we set pin number 26 as an input pull-down
pin and assign a variable button_pin
to hold the pin data. We also set pin number 15 as an output pin and assign a variable led_pin
for that pin.
button_pin = Pin(26, Pin.IN, Pin.PULL_DOWN)
led_pin = Pin(15, Pin.OUT)
In the while loop, we check if the button_pin value is 1 then toggle the led_pin. We use 0.2s delay to avoid button press conflict.
Conclusion
This is just a beginner guide to Raspberry Pi Pico. Hope you find it useful and informative. Stay tuned for more advanced Raspberry Pi Pico projects.
Let me know about your projects and thoughts related to Raspberry Pi Pico in the comment section below.