Led blinking is a very simple and basic project to start with Arduino. LED blinking is nothing but turning ON and OFF an LED light.
Though Arduino LED blinking is very basic, it can be used in further stages in creating various types of projects which will be very interesting. It has a wide range of usage in our day-to-day lives; for e.g. in Traffic signals, decorating purposes, and many more.
Projects with LED can be as easy as LED blinking or as challenging as sound/music-sensitive lights or LED Cube 8x8x8.
In this tutorial, we are going to show you how to control LEDs using Arduino through three simple Arduino LED projects.
- Arduino onboard LED Blinking.
- LED Blinking Arduino – Blink an External LED.
- Control Multiple LEDs using Arduino.
Table of Contents
After complete reading of this post, you will have complete knowledge of how LED works with Arduino. And you will be able to do your own Arduino LED projects.
You will need :
- LED
- Cables
- 330 Ω Resistor
- Arduino board (it can be of any kind, but I used here UNO board).
- Breadboard/Circuit-board Holder.
- USB cable
- Computer with Arduino software installed (you can download the Arduino IDE from here..)
Before starting you should know the basics of an Arduino and LED.
What is Arduino?
Arduino is a microcontroller development board. It has a microcontroller as its heart and some digital and analog Input Output pins. We can give some information/data in the form of voltage to Arduino via digital or analog input pins. And in the output pin, Arduino generates some control signal.
Arduino also has a nice and user-friendly GUI for programming the Arduino board. We can control the output signal according to the input signal by programming the Arduino board using the Arduino GUI.
We can download the Arduino GUI from Arduino’s official website and can learn more about Arduino from there.
LED (Light Emitting Diode)
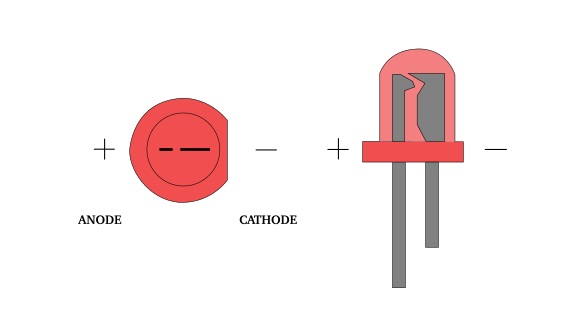
A light-emitting diode (LED) is a semiconductor light source. It emits light when current flows through it.
LEDs have two leads. One lead is shorter, that is the cathode(-ve) and another one is longer, that is the anode(+ve). You can also identify the cathode by spotting the flat side of the LED and another one is the anode. LEDs have polarities. So the long leg should connect to a digital pin on the Arduino board. And shorter legs should go to the GND.
Arduino onboard LED Blinking
Most Arduinos have an onboard LED that you can control. In this section, you are going to learn how to control that LED. So for this project, you only need an Arduino board and a PC or Mac.
In this section, I am using an Arduino Uno board. But you can use any Arduino board which has an on-board LED. On the Arduino Uno, mega, and zero this LED is connected to pin no 13. You can see it in the picture below.
Here I am going to turn on this LED for one second, then off for one second, repeatedly.
Circuit Diagram
In this project, we don’t need an external component. Just connect the Arduino board to pc via a USB cable and upload the code.
Arduino Code
int ledPin =13;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
digitalWrite(ledPin, HIGH);
delay(1000);
digitalWrite(ledPin, LOW);
delay(1000);
}
Explaining the code
The Arduino program has mainly two parts one is setup and one is loop. Setup parts only run once and the loop runs continuously.
So the first thing we do is define a variable that will hold the number of the pin which is connected to the LED. Then we set this pin as the output pin inside the setup()
function like this
void setup() {
pinMode(ledPin, OUTPUT);
}
Finally, we have to turn the LED on and off using the sketch’s loop() function. Inside the loop, we call digitalWrite()
function two times. digitalWrite()
with HIGH value will turn the led on and with LOW value turn the led off.
If we only use these two lines of code the led will blink so fast that we can’t understand it with the naked eye. So we have to put some delay in between to slow things down. We are using the delay()
function for that. It works with milliseconds.
I will use delay(1000)
; after digitalWrite(led, HIGH)
; to hold the HIGH state i.e LED on state for 1 second. And we will use the same after digitalWrite(led, LOW)
; to hold the LOW state i.e LED off state for one second.
LED Blinking Arduino – Blink an External LED
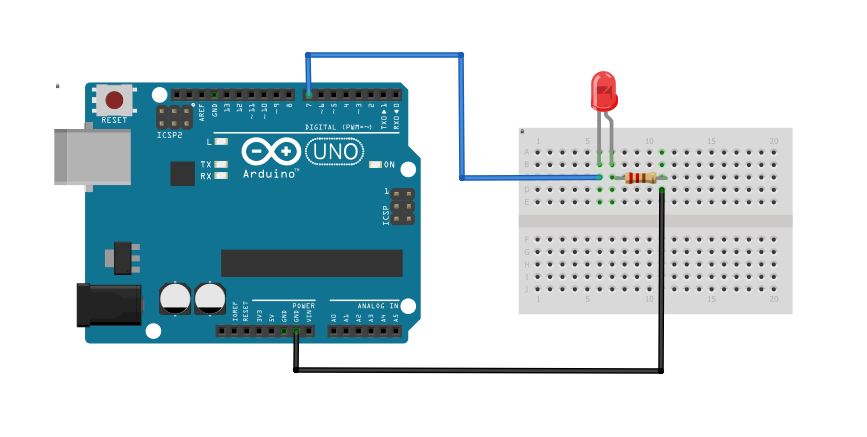
In this Arduino LED project, we will turn on an external LED for one second, then off for one second, repeatedly.
Circuit Diagram
Connect the +ve lead of the LED to a digital pin on the Arduino and -ve lead to Ground.
We need to use a resistor in series with the LED to limit the current through the LED and to prevent that it burns.
Here we are using an Arduino Uno board and using digital pin 7 to control the LED. So we will Code the Arduino board accordingly.
Arduino Code
int ledPin = 7;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
digitalWrite(ledPin, HIGH);
delay(1000);
digitalWrite(ledPin, LOW);
delay(1000);
}
Explaining the Code
Here we are going to write a program to blink an LED interval of 1000 ms (1 sec). The code will be the same as onboard LED Blinking. You only have to change the pin number accordingly. We are using pin no 7 (Digital Pin) so we will write int ledPin = 7
; and other things will remain the same.
First, we initialize pin no 7 as the led pin and set it as an output pin. Then we create an infinite loop in which the LED pin is in a high state for 1000 ms and low for the next 1000 ms and continues.
Control Multiple LEDs using Arduino
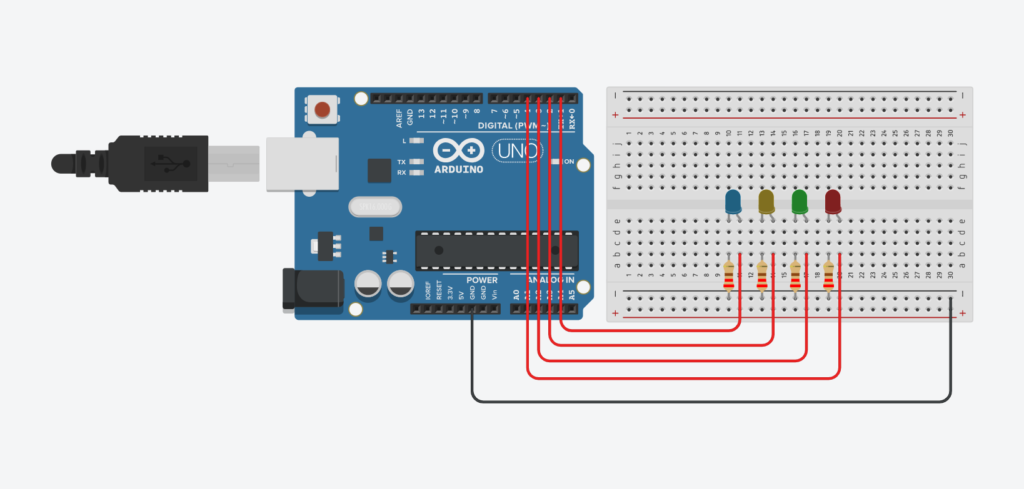
In this Arduino LED project, we will learn how to control multiple LEDs. Here we will take four different color LEDs and turn them on and off simultaneously.
Circuit Diagram
Take four different color LEDs ( you can use the same color led as well ) and connect their +ve leg to four different digital pins of the Arduino. Here I am using pins 1,2,3 and 4 and an Arduino Uno board. So connect their +ve leg to the Arduino’s pins no 1,2,3 and 4.
Connect their -ve leg to the ground with a series connection of a 220-ohm resistor.
Arduino Code
int led_red = 1;
int led_green = 2;
int led_yellow = 3;
int led_blue = 4;
void setup() {
pinMode(led_red, OUTPUT);
pinMode(led_green, OUTPUT);
pinMode(led_yellow, OUTPUT);
pinMode(led_blue, OUTPUT);
}
void loop() {
digitalWrite(led_red, HIGH);
delay(1000);
digitalWrite(led_red, LOW);
digitalWrite(led_green, HIGH);
delay(1000);
digitalWrite(led_green, LOW);
digitalWrite(led_yellow, HIGH);
delay(1000);
digitalWrite(led_yellow, LOW);
digitalWrite(led_blue, HIGH);
delay(1000);
digitalWrite(led_blue, LOW);
}
Explaining the Code
Take four Integer Variables to hold the four pin numbers we are using and assign their value. Inside the setup() function I set those four pins as output pins like this pinMode(led_red, OUTPUT)
;
Then in the loop()
section, I turn the LED on for one second and then turn it off for one second by these lines of code
digitalWrite(led_red, HIGH);
delay(1000);
digitalWrite(led_red, LOW);
delay(1000);
Repeat this for all four LEDs and upload the code to the Arduino.
You can optimize this code like this:-
// assign LED pin to the array
int ledPin[4]={1,2,3,4};
int delay_t = 500;
void setup() {
// set pins 1,2,3,4 as output
for (int i = 0; i <= 4; i++) {
pinMode(ledPin[i], OUTPUT);
}
}
void loop() {
// iterate over the pins:
for (int i = 0; i <= 3; i++) {
digitalWrite(ledPin[i], HIGH);
delay(delay_t);
digitalWrite(ledPin[i], LOW);
delay(delay_t);
}
}
Very useful and benefit tutorial. Please upload multiple led next by next loop blinking also